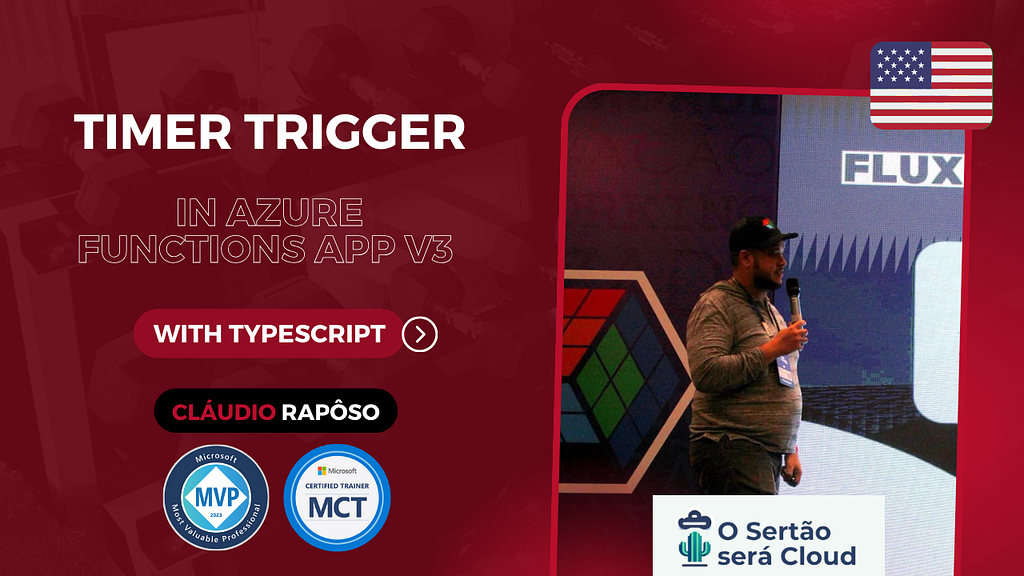
In the world of modern applications, the ability to execute scheduled tasks is crucial to keep systems running efficiently and smoothly. The Azure Functions App offers a powerful and flexible solution for this through the TimerTrigger. In this article, we will explore how TimerTrigger works in the Azure Functions App using TypeScript and how you can apply it to solve everyday problems with practical examples.
What are Triggers?
Triggers are integrated components of Azure Functions that listen for specific events or conditions. When these events or conditions are met, the trigger is activated, and the associated function is executed. Azure Functions supports a variety of triggers, allowing you to respond to different types of events.
Types of Triggers:
- HTTP Trigger: Executes a function in response to an HTTP request. Useful for creating APIs or webhooks.
- Timer Trigger: Executes a function at a scheduled time or interval. Ideal for periodic tasks like data cleaning or scheduled reports.
- Blob Trigger: Executes a function when a new blob is detected or an existing blob is updated in Azure Blob Storage. Useful for processing files as they are uploaded.
- Queue Trigger: Executes a function when a new message is added to an Azure Storage queue. Useful for processing background tasks or messages.
- Event Grid Trigger: Executes a function in response to events published in Azure Event Grid. Useful for handling events from various Azure services or custom events.
- Service Bus Trigger: Executes a function when a new message is received in an Azure Service Bus queue or topic. Useful for message-based applications.
Each type of trigger has its specific use cases and settings, allowing you to adapt your functions to efficiently respond to various events.
What is TimerTrigger in detail?
The TimerTrigger is a type of trigger that allows you to execute functions at regular intervals or specific times. It’s ideal for tasks that need to be executed periodically, like data cleaning, sending reports, or cache updates.
Using TimerTrigger, you define a CRON expression to schedule the execution of the function. The Azure Functions App interprets this expression and triggers the function as per the defined schedule.
Setting Up TimerTrigger
To get started, you need to have an Azure Functions project configured for TypeScript. Let’s go through the basic steps to set it up.
Creating a New Project
First, create a new Azure Functions project with TypeScript support:
func init MyFunctionApp --typescript
cd MyFunctionApp
func new --template "TimerTrigger" --name MyTimerFunction
This will create a new project with a basic TimerTrigger function.
Configuring the Function
In the function.json file of the created function, you’ll find the basic TimerTrigger configuration:
{
"bindings": [
{
"name": "myTimer",
"type": "timerTrigger",
"direction": "in",
"schedule": "0 */5 * * * *"
}
]
}
he expression 0 */5 * * * * indicates that the function will run every 5 minutes.
Implementing the Function in TypeScript
In the index.ts file, you’ll find a basic implementation example:
import { AzureFunction, Context } from "@azure/functions";
const timerTrigger: AzureFunction = async function (context: Context, myTimer: any): Promise<void> {
const currentTime = new Date().toISOString();
context.log(`Timer trigger function ran at ${currentTime}`);
};
export default timerTrigger;
This code logs the time when the function is executed.
Use Cases
Here are five examples of how you can use TimerTrigger for different tasks, applying best coding practices:
Cleaning Old Data
This example cleans up old data from a database table. Suppose you’re using MongoDB and want to remove documents older than 30 days.
import { AzureFunction, Context } from "@azure/functions";
import { MongoClient } from "mongodb";
const uri = process.env["MONGO_DB_CONNECTION_STRING"];
const client = new MongoClient(uri);
const timerTrigger: AzureFunction = async function (context: Context, myTimer: any): Promise<void> {
const currentTime = new Date();
const cutoffDate = new Date(currentTime.setDate(currentTime.getDate() - 30));
try {
await client.connect();
const database = client.db("mydatabase");
const collection = database.collection("mycollection");
const result = await collection.deleteMany({ createdAt: { $lt: cutoffDate } });
context.log(`Deleted ${result.deletedCount} old documents.`);
} catch (err) {
context.log(`Error cleaning old data: ${err.message}`);
} finally {
await client.close();
}
};
export default timerTrigger;
Sending Daily Reports
In this example, we send a daily report via email using the nodemailer library.
import { AzureFunction, Context } from "@azure/functions";
import nodemailer from "nodemailer";
const transporter = nodemailer.createTransport({
service: "gmail",
auth: {
user: process.env["EMAIL_USER"],
pass: process.env["EMAIL_PASS"],
},
});
const timerTrigger: AzureFunction = async function (context: Context, myTimer: any): Promise<void> {
const mailOptions = {
from: process.env["EMAIL_USER"],
to: "recipient@example.com",
subject: "Daily Report",
text: "Here is your daily report...",
};
try {
await transporter.sendMail(mailOptions);
context.log("Daily report sent successfully.");
} catch (err) {
context.log(`Error sending daily report: ${err.message}`);
}
};
export default timerTrigger;
Updating Cache
Here, we update the cache of an application, simulating the update of a cache key in a Redis service.
import { AzureFunction, Context } from "@azure/functions";
import redis from "redis";
const client = redis.createClient({ url: process.env["REDIS_URL"] });
const timerTrigger: AzureFunction = async function (context: Context, myTimer: any): Promise<void> {
const cacheKey = "myCacheKey";
const cacheValue = "Updated cache value";
try {
await new Promise((resolve, reject) => {
client.set(cacheKey, cacheValue, (err) => {
if (err) reject(err);
resolve("Cache updated");
});
});
context.log("Cache updated successfully.");
} catch (err) {
context.log(`Error updating cache: ${err.message}`);
}
};
export default timerTrigger;
Data Backup
This example backs up data from a database to a storage service like Azure Blob Storage.
import { AzureFunction, Context } from "@azure/functions";
import { BlobServiceClient } from "@azure/storage-blob";
import { MongoClient } from "mongodb";
const mongoUri = process.env["MONGO_DB_CONNECTION_STRING"];
const blobServiceClient = BlobServiceClient.fromConnectionString(process.env["AZURE_STORAGE_CONNECTION_STRING"]);
const client = new MongoClient(mongoUri);
const backupData: AzureFunction = async function (context: Context, myTimer: any): Promise<void> {
const currentTime = new Date().toISOString();
const containerClient = blobServiceClient.getContainerClient("backups");
const blobClient = containerClient.getBlockBlobClient(`backup-${currentTime}.json`);
try {
await client.connect();
const database = client.db("mydatabase");
const collection = database.collection("mycollection");
const data = await collection.find({}).toArray();
await blobClient.upload(JSON.stringify(data), Buffer.byteLength(JSON.stringify(data)));
context.log("Backup completed successfully.");
} catch (err) {
context.log(`Error during backup: ${err.message}`);
} finally {
await client.close();
}
};
export default backupData;
System Status Check
In this example, we check the status of an API service and log the result.
import { AzureFunction, Context } from "@azure/functions";
import axios from "axios";
const checkSystemStatus: AzureFunction = async function (context: Context, myTimer: any): Promise<void> {
const apiUrl = "https://api.example.com/health";
try {
const response = await axios.get(apiUrl);
if (response.status === 200) {
context.log("System is up and running.");
} else {
context.log(`System returned status code ${response.status}.`);
}
} catch (err) {
context.log(`Error checking system status: ${err.message}`);
}
};
export default checkSystemStatus;
Conclusion
The examples above illustrate how TimerTrigger in Azure Functions App can be used in various day-to-day scenarios, from cleaning old data to sending reports and backups. Each example is designed to be clear and straightforward, keeping the code efficient and easy to maintain. By implementing these functions, you can automate important tasks and ensure that your system operates continuously and efficiently.
TimerTrigger in Azure Functions App V3 with TypeScript was originally published in Stackademic on Medium, where people are continuing the conversation by highlighting and responding to this story.